JavaScript has cemented its place as one of the most popular programming languages for web development, enabling dynamic and interactive user experiences js. Whether you’re a budding developer or a seasoned programmer, refining your JavaScript skills is crucial to staying competitive. Here are ten essential tips to help you master JavaScript and elevate your coding game.
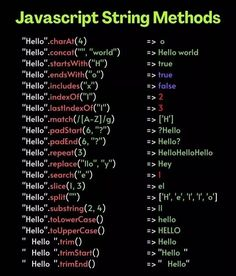
1. Understand the Basics of Scopes and Closures
JavaScript’s scope and closures are foundational.
- Scope: Understand the difference between function scope and block scope introduced by
let
andconst
in ES6. - Closures: Master how inner functions retain access to variables in their outer functions, even after the outer function has executed.
📌 Example:
javascriptCopy codefunction outerFunction(outerVar) {
return function innerFunction(innerVar) {
console.log(`Outer: ${outerVar}, Inner: ${innerVar}`);
};
}
const myFunction = outerFunction('Hello');
myFunction('World'); // Outer: Hello, Inner: World
2. Learn Asynchronous Programming
Asynchronous programming is at JavaScript’s core with its event-driven model. Familiarize yourself with:
- Callbacks
- Promises
async
/await
📌 Tip: Always handle errors in asynchronous code using .catch()
for promises or try-catch
with async/await
.
3. Avoid Common Pitfalls with this
Understanding this
can save you hours of debugging.
- In global functions,
this
refers to the global object (window
in browsers). - In strict mode (
'use strict';
), it’sundefined
. - Arrow functions do not have their own
this
context; they inherit from the surrounding scope.
📌 Example:
javascriptCopy codeconst obj = {
value: 42,
regularFunction() {
console.log(this.value);
},
arrowFunction: () => {
console.log(this.value);
},
};
obj.regularFunction(); // 42
obj.arrowFunction(); // undefined
4. Use Modern JavaScript Features
ES6 and beyond introduced features like:
- Destructuring
- Template literals
- Modules (
import
/export
) - Rest/Spread operators
📌 Example:
javascriptCopy codeconst person = { name: 'Alice', age: 25 };
const { name, age } = person;
console.log(`${name} is ${age} years old.`); // Alice is 25 years old.
5. Optimize with Array and Object Methods
JavaScript provides powerful methods like map()
, filter()
, and reduce()
for arrays, and Object.keys()
, Object.values()
, and Object.entries()
for objects.
📌 Example:
javascriptCopy codeconst numbers = [1, 2, 3, 4, 5];
const squares = numbers.map(n => n ** 2);
console.log(squares); // [1, 4, 9, 16, 25]
6. Write Clean and Readable Code
- Follow consistent coding conventions.
- Use meaningful variable and function names.
- Break large functions into smaller, reusable ones.
- Comment your code where necessary.
7. Debug Like a Pro
Make use of browser developer tools:
- Use
console.log()
intelligently to inspect variables. - Leverage breakpoints in the debugger for step-by-step execution.
- Use
console.error()
andconsole.table()
for better insights.
8. Master Error Handling
Graceful error handling ensures your application doesn’t crash unexpectedly.
- Use
try-catch
blocks effectively. - Create custom error classes for better debugging.
📌 Example:
javascriptCopy codetry {
throw new Error('Something went wrong!');
} catch (error) {
console.error(error.message);
}
9. Avoid Common Anti-Patterns
Stay clear of practices that lead to bugs or poor performance:
- Declaring variables without
let
,const
, orvar
. - Modifying global objects unnecessarily.
- Overusing
eval()
orwith
.
10. Keep Learning and Experimenting
JavaScript evolves rapidly. Stay updated with:
- New features in the ECMAScript specification.
- Popular frameworks like React, Vue, or Angular.
- Tools like TypeScript for enhanced productivity and maintainability.
Conclusion
Mastering JavaScript takes time, practice, and a commitment to learning. By understanding its quirks, leveraging modern features, and avoiding common pitfalls, you’ll set yourself apart as a proficient developer.